Added restart and level feature
This commit is contained in:
parent
a57e318e31
commit
f3306ade87
21
LICENSE
21
LICENSE
@ -1,21 +0,0 @@
|
|||||||
MIT License
|
|
||||||
|
|
||||||
Copyright (c) 2020 Logan Hunt
|
|
||||||
|
|
||||||
Permission is hereby granted, free of charge, to any person obtaining a copy
|
|
||||||
of this software and associated documentation files (the "Software"), to deal
|
|
||||||
in the Software without restriction, including without limitation the rights
|
|
||||||
to use, copy, modify, merge, publish, distribute, sublicense, and/or sell
|
|
||||||
copies of the Software, and to permit persons to whom the Software is
|
|
||||||
furnished to do so, subject to the following conditions:
|
|
||||||
|
|
||||||
The above copyright notice and this permission notice shall be included in all
|
|
||||||
copies or substantial portions of the Software.
|
|
||||||
|
|
||||||
THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
|
|
||||||
IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
|
|
||||||
FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE
|
|
||||||
AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER
|
|
||||||
LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM,
|
|
||||||
OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE
|
|
||||||
SOFTWARE.
|
|
14
README.md
14
README.md
@ -1,14 +0,0 @@
|
|||||||
# Asteroids-CPP
|
|
||||||
The game asteroids in c++ using the simplified drawing library provided by my school.
|
|
||||||
|
|
||||||
## Requirements
|
|
||||||
|
|
||||||
* libglut-dev
|
|
||||||
* g++
|
|
||||||
* make
|
|
||||||
|
|
||||||
## Running
|
|
||||||
|
|
||||||
All that is needed to run is a simple ```make```, and then run the game with ```./a.out```
|
|
||||||
|
|
||||||
[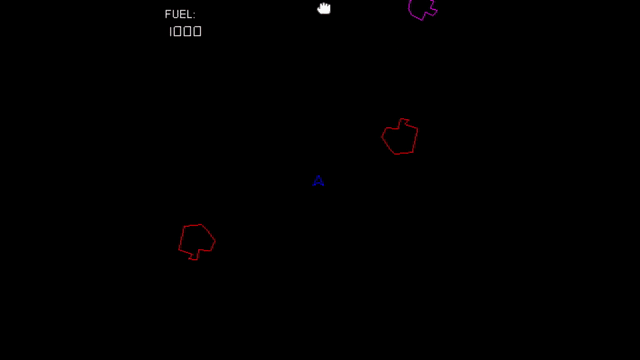](https://postimg.cc/zbCRV6dR)
|
|
BIN
UML Diagram.pdf
BIN
UML Diagram.pdf
Binary file not shown.
29
src/game.cpp
29
src/game.cpp
@ -26,7 +26,7 @@ Game :: Game ( const Point &tl , const Point &br ) : topLeft ( tl ) , bottomRigh
|
|||||||
vector<Rock *> Game :: createRocks()
|
vector<Rock *> Game :: createRocks()
|
||||||
{
|
{
|
||||||
vector<Rock *> initRocks;
|
vector<Rock *> initRocks;
|
||||||
for ( int i = 0; i < random ( 5 , 8 ); i++ )
|
for ( int i = 0; i < random ( 5 + 2 * this->level , 8 + 2 * this->level ); i++ )
|
||||||
{
|
{
|
||||||
Point rockPoint = Point ( random( -190 , 190 ) , random ( -190 , 190 ) );
|
Point rockPoint = Point ( random( -190 , 190 ) , random ( -190 , 190 ) );
|
||||||
Velocity velocity;
|
Velocity velocity;
|
||||||
@ -136,6 +136,7 @@ void Game :: cleanUpZombies()
|
|||||||
bullets.erase( bullets.begin() + i );
|
bullets.erase( bullets.begin() + i );
|
||||||
}
|
}
|
||||||
}
|
}
|
||||||
|
if (rocks.size() > 0) {
|
||||||
for ( int i = 0; i < rocks.size(); i++ )
|
for ( int i = 0; i < rocks.size(); i++ )
|
||||||
{
|
{
|
||||||
if ( !rocks[i]->isAlive() )
|
if ( !rocks[i]->isAlive() )
|
||||||
@ -146,6 +147,7 @@ void Game :: cleanUpZombies()
|
|||||||
}
|
}
|
||||||
}
|
}
|
||||||
}
|
}
|
||||||
|
}
|
||||||
|
|
||||||
// Handle all collisions
|
// Handle all collisions
|
||||||
void Game :: handleCollisions()
|
void Game :: handleCollisions()
|
||||||
@ -209,6 +211,14 @@ void Game :: draw ( const Interface &ui )
|
|||||||
drawBullets();
|
drawBullets();
|
||||||
drawNumber ( Point ( -170 , 170 ) , (int) ship->getFuel() );
|
drawNumber ( Point ( -170 , 170 ) , (int) ship->getFuel() );
|
||||||
drawText ( Point ( -170 , 180 ) , "FUEL:" );
|
drawText ( Point ( -170 , 180 ) , "FUEL:" );
|
||||||
|
drawNumber ( Point (-170, 130) , (int) level);
|
||||||
|
drawText ( Point(-170, 140), "LEVEL:");
|
||||||
|
if (!ship->isAlive()) {
|
||||||
|
drawText( Point ( -90 , 0 ) , "Play again? (space to continue)");
|
||||||
|
}
|
||||||
|
if (rocks.size() == 0 && ship->isAlive()) {
|
||||||
|
drawText( Point ( -90 , 0 ) , "Press space to continue to next level ");
|
||||||
|
}
|
||||||
}
|
}
|
||||||
|
|
||||||
// Draw rocks
|
// Draw rocks
|
||||||
@ -241,7 +251,7 @@ void Game :: drawBullets()
|
|||||||
// Handle in-game input
|
// Handle in-game input
|
||||||
void Game::handleInput(const Interface & ui)
|
void Game::handleInput(const Interface & ui)
|
||||||
{
|
{
|
||||||
if ( ship->isAlive() )
|
if ( ship->isAlive() && rocks.size() > 0 )
|
||||||
{
|
{
|
||||||
if ( ui.isUp() )
|
if ( ui.isUp() )
|
||||||
{
|
{
|
||||||
@ -274,5 +284,18 @@ void Game::handleInput(const Interface & ui)
|
|||||||
ship->setThrusting ( false );
|
ship->setThrusting ( false );
|
||||||
}
|
}
|
||||||
}
|
}
|
||||||
|
else if ( !ship->isAlive() && rocks.size() > 0){
|
||||||
|
if ( ui.isSpace() ) {
|
||||||
|
rocks = createRocks();
|
||||||
|
ship = new Ship ( Point() );
|
||||||
|
level = 0;
|
||||||
|
}
|
||||||
|
}
|
||||||
|
else if ( ship->isAlive() && rocks.size() == 0) {
|
||||||
|
if ( ui.isSpace() ) {
|
||||||
|
rocks = createRocks();
|
||||||
|
ship = new Ship ( Point() );
|
||||||
|
level++;
|
||||||
|
}
|
||||||
|
}
|
||||||
}
|
}
|
||||||
|
@ -26,6 +26,7 @@ private:
|
|||||||
vector<Rock *> rocks;
|
vector<Rock *> rocks;
|
||||||
vector<Bullet *> bullets;
|
vector<Bullet *> bullets;
|
||||||
Ship* ship;
|
Ship* ship;
|
||||||
|
int level = 0;
|
||||||
|
|
||||||
float getClosestDistance( const FlyingObject &obj1 , const FlyingObject &obj2 ) const;
|
float getClosestDistance( const FlyingObject &obj1 , const FlyingObject &obj2 ) const;
|
||||||
|
|
||||||
|
Loading…
Reference in New Issue
Block a user